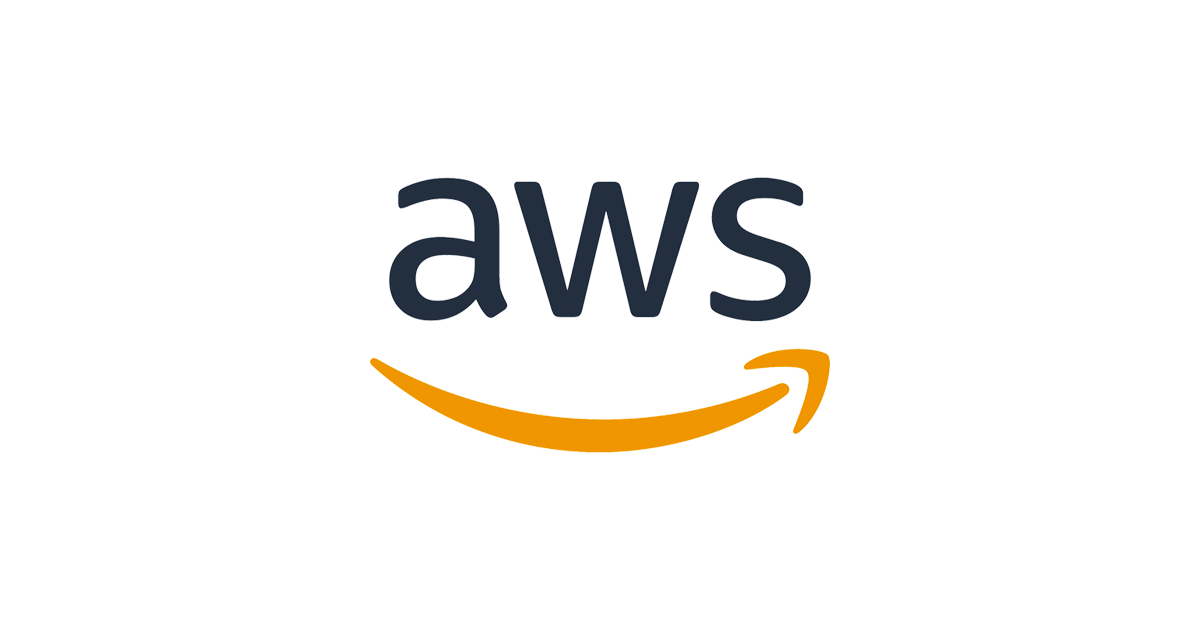
Amazon Location Service の経路計算を試してみた
こんにちは!DA(データアナリティクス)事業本部 インテグレーション部の大高です。
Amazon Location Serviceでは、ある座標からある座標までの経路計算をすることができます。
今回は、boto3を利用してこの「経路計算」を試してみたいと思います!
前提
試した環境は、以下のような環境となります。
- Python 3.7
boto3
の1.17.86
がインストール済みboto3
でアクセスできるように、~/.aws/config
および~/.aws/credentials
は設定済み
また、前提条件として下記のドキュメントに記載のとおり「calculator」が作成されている必要があります。今回は事前に検証環境にexplore.calculator
という名前の「calculator」を作成済みです。
認証に関しては、boto3を利用するので特に準備はしていません。
ルート計算を試してみる
では、早速ためしてみましょう。弊社の秋葉原オフィスの座標 139.77474719732845,35.69727421514524
から、同じく弊社のカフェの座標139.7746728170799,35.696761744911285
までのルートを計算してみます。
import boto3 import json location = boto3.client('location') res = location.calculate_route( CalculatorName='explore.calculator', DeparturePosition=[ 139.77474719732845, 35.69727421514524 ], DestinationPosition=[ 139.7746728170799, 35.696761744911285 ], IncludeLegGeometry=True, ) print(json.dumps(res, ensure_ascii=False, indent=2))
結果は、以下のように出力されます。カーナビでよくあるような「次は〜に向かう」という指示に関する情報がLegs.Steps
に表示されますが、今回はオプションとしてIncludeLegGeometry=True
を指定したので、Legs.Gemetry
にLineString
として実際の経路そのものが表示されています。
なお、Legs
の詳細については、boto3のドキュメントによる解説がわかりやすかったです。(Response Structure > Legs の箇所)
{ "ResponseMetadata": { "RequestId": "c1a91f90-ceb9-455d-9beb-cbb584d470e0", "HTTPStatusCode": 200, "HTTPHeaders": { (snip...) }, "RetryAttempts": 0 }, "Legs": [ { "Distance": 0.5068328441359271, "DurationSeconds": 86.742858203, "EndPosition": [ 139.77466498245855, 35.6965482129957 ], "Geometry": { "LineString": [ [ 139.77475213305382, 35.697539190600715 ], (snip...) [ 139.77466498245855, 35.6965482129957 ] ] }, "StartPosition": [ 139.77475213305382, 35.697539190600715 ], "Steps": [ { "Distance": 0.07915825507094484, "DurationSeconds": 14.998406223, "EndPosition": [ 139.77562621100483, 35.697514435076606 ], "GeometryOffset": 1, "StartPosition": [ 139.77475214287932, 35.69753916855547 ] }, { "Distance": 0.20835252733608864, "DurationSeconds": 33.410650263, "EndPosition": [ 139.7754485204856, 35.69564334740039 ], "GeometryOffset": 6, "StartPosition": [ 139.77559340660127, 35.696670763094865 ] }, { "Distance": 0.13482359376608183, "DurationSeconds": 21.619819044, "EndPosition": [ 139.77555995407374, 35.6964099282988 ], "GeometryOffset": 10, "StartPosition": [ 139.77544852301781, 35.69564331908335 ] }, { "Distance": 0.0844984679628118, "DurationSeconds": 16.713982673, "EndPosition": [ 139.7746649770672, 35.6965481649428 ], "GeometryOffset": 14, "StartPosition": [ 139.7755600030956, 35.696409917885546 ] } ] } ], "Summary": { "DataSource": "Esri", "Distance": 0.5068328441359271, "DistanceUnit": "Kilometers", "DurationSeconds": 86.742858203, "RouteBBox": [ 139.7746649770672, 35.69562691684848, 139.77591169088817, 35.697539190600715 ] } }
地図で確認がしたい
さて、当然なのですがこの経路がどのような経路なのか、座標では想像がつかないので地図で確認したいです。簡単そうな方法としては、GeoJSON形式のデータを用意し、以下のサービスを利用して表示するのが良さそうでした。
まずは、先程のコードを少し修正してGeoJSONを作成してみます。
import boto3 import json location = boto3.client('location') res = location.calculate_route( CalculatorName='explore.calculator', DeparturePosition=[ 139.77474719732845, 35.69727421514524 ], DestinationPosition=[ 139.7746728170799, 35.696761744911285 ], IncludeLegGeometry=True, ) leg_geometry = res['Legs'][0]['Geometry'] geojson = { "type": "FeatureCollection", "features": [ { "type": "Feature", "geometry":{ "type": "LineString", "coordinates": leg_geometry['LineString'] }, "properties": {} } ] } print(json.dumps(geojson, ensure_ascii=False, indent=2))
これで、以下のようにGeoJSON形式で出力されます。
{ "type": "FeatureCollection", "features": [ { "type": "Feature", "geometry": { "type": "LineString", "coordinates": [ [ 139.77475213305382, 35.697539190600715 ], [ 139.77475214287932, 35.69753916855547 ], [ 139.77482217767738, 35.69753780170532 ], [ 139.77535752731862, 35.69751925159617 ], [ 139.77547618292908, 35.6975171687769 ], [ 139.77562621100483, 35.697514435076606 ], [ 139.77559340660127, 35.696670763094865 ], [ 139.7755599513167, 35.69640995506903 ], [ 139.77554094559085, 35.696287394172444 ], [ 139.7754485204856, 35.69564334740039 ], [ 139.77544852301781, 35.69564331908335 ], [ 139.77591169088817, 35.69562691684848 ], [ 139.77582954953735, 35.69582745369623 ], [ 139.77555995407374, 35.6964099282988 ], [ 139.7755600030956, 35.696409917885546 ], [ 139.77531052052947, 35.69652154392283 ], [ 139.7747489406754, 35.69654504071551 ], [ 139.7746649770672, 35.6965481649428 ], [ 139.77466498245855, 35.6965482129957 ] ] }, "properties": {} } ] }
出力されたGeoJSONを先程のサイトを利用して確認してみましょう。
経路が地図で表示されました!
秋葉原オフィスのある「産報佐久間ビル」から、「DevelopersIO CAFE」までの経路がバッチリ出ていますね。経路は「車」を利用した経路になっているので、きちんと一方通行も考慮した経路になっているのが分かります。
徒歩の経路も確認してみる
ついでに徒歩の経路も確認してみましょう。以下のようにTravelMode='Walking'
を指定することで「徒歩」を指定しています。なお、デフォルトはTravelMode='Car'
なので先程は「車」を利用した経路になっていました。
import boto3 import json location = boto3.client('location') res = location.calculate_route( CalculatorName='explore.calculator', DeparturePosition=[ 139.77474719732845, 35.69727421514524 ], DestinationPosition=[ 139.7746728170799, 35.696761744911285 ], IncludeLegGeometry=True, TravelMode='Walking', )
{ "type": "FeatureCollection", "features": [ { "type": "Feature", "geometry": { "type": "LineString", "coordinates": [ [ 139.77475213305382, 35.697539190600715 ], [ 139.7747521270913, 35.69753917336058 ], [ 139.7748221617526, 35.697537806513104 ], [ 139.7753575754365, 35.69751925644018 ], [ 139.77532535688877, 35.69676736015084 ], [ 139.77531051683042, 35.696521522868565 ], [ 139.77531052486535, 35.696521556544575 ], [ 139.77474894599823, 35.69654505329597 ], [ 139.77466498253756, 35.69654817751776 ], [ 139.77466498245855, 35.6965482129957 ] ] }, "properties": {} } ] }
これを地図で表示すると、以下のようになります。
今度は徒歩なので、回り道をせずに最短ルートでの経路になっていることがわかりますね。
まとめ
以上、Amazon Location Service の経路計算を試してみました!
日本の地図にどこまで対応しているか少し気になっていましたが、試してみた限りでは日本の道路にもきちんと対応していて、とても良さそうです。
どなたかのお役に立てば幸いです。それでは!